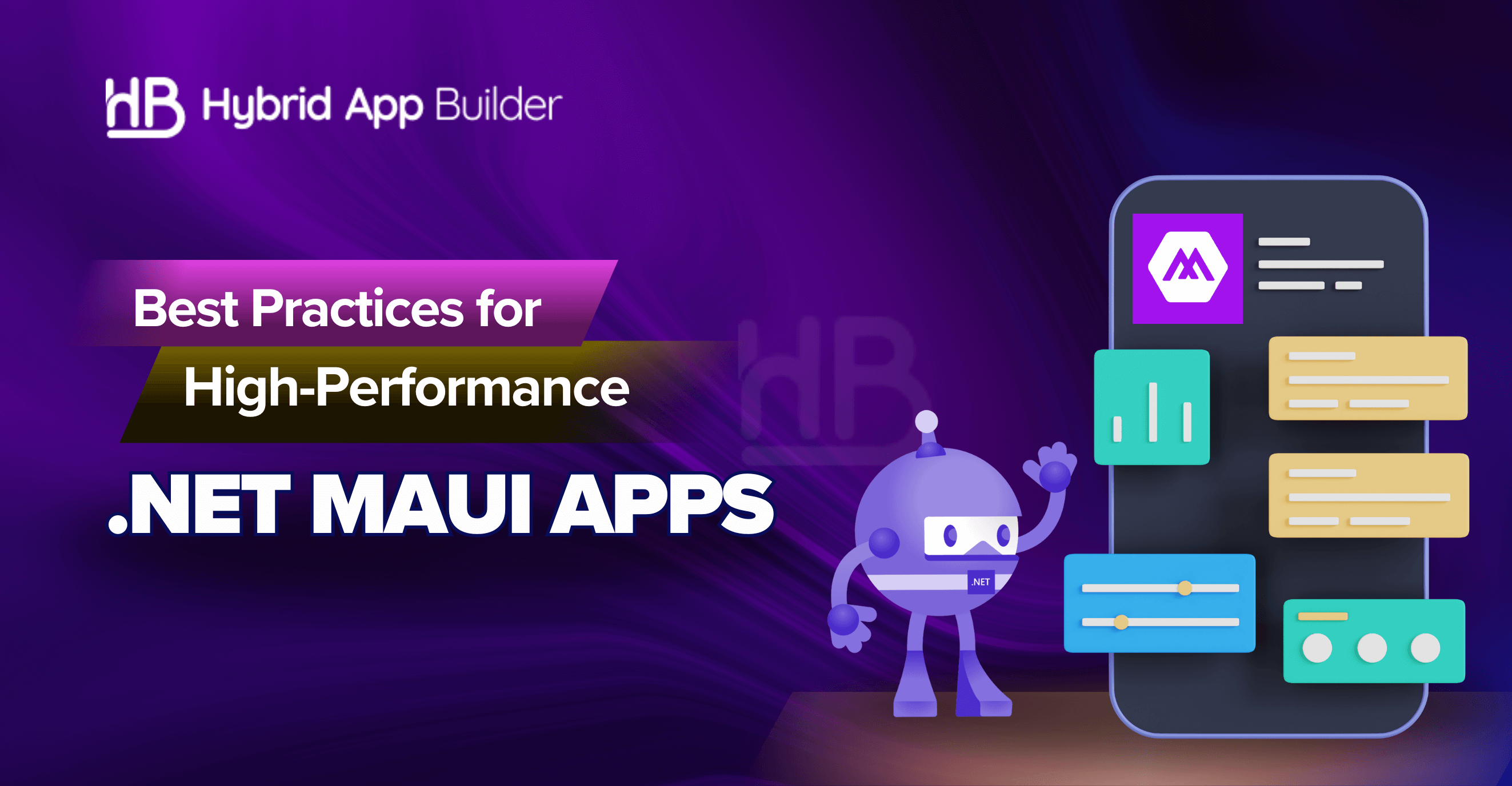
With mobile and cross-platform applications becoming business-critical, .NET MAUI (Multi-platform App UI) has emerged as a powerful successor to Xamarin, enabling you to build high-performance, cross-platform apps using a single codebase. Whether you’re planning a new app or navigating the path of .NET MAUI migration, delivering speed and responsiveness is key to user retention and satisfaction.
In this blog, we’ll walk through proven MAUI best practices that help developers and business owners build high-performance .NET MAUI apps that scale, perform well, and deliver seamless experiences on Android, iOS, macOS, and Windows.
Also go through a detailed comparison between Xamarin and .NET MAUI: The Future of Cross-Platform Development
Why High Performance Matters in .NET MAUI Apps
Performance isn’t just a developer’s concern — it’s a business priority. A sluggish or memory-intensive app frustrates users, increases churn, and reflects poorly on your brand. On the flip side, a high-performance MAUI app:
- Loads faster and runs smoother on all platforms
- Saves device resources (battery, memory)
- Boosts user engagement and reviews
- Enables long-term maintainability and scalability
Whether you’re building a new project or modernizing high performance Xamarin apps, adopting best practices from day one will future-proof your investment.
Optimize Your App Startup Time
First impressions matter. The faster your app launches, the better the user experience. Here’s how to minimize startup time in your .NET MAUI app:
Use App Lifecycle Events Efficiently
Avoid doing too much work in the constructor or OnStart. Offload non-essential logic to background threads or defer it until after the UI is fully loaded.
Remove Unused Resources
Strip out unused images, fonts, or heavy libraries. Every unnecessary asset slows down your app’s initialization.
Use AOT (Ahead-of-Time) Compilation
Enable AOT where applicable (especially on iOS) to improve performance and reduce startup time by compiling IL to native code before runtime.
Minimize UI Thread Load
.NET MAUI apps share a single UI thread across platforms. Overloading this thread leads to UI freezes, stutters, or delays.
Use Async and Await
Move blocking or time-consuming operations off the main thread using async/await. This keeps the UI responsive, especially during network calls or data loading.
var result = await GetDataAsync();
Leverage Data Virtualization
If you’re working with large lists or datasets, use UI controls like CollectionView with incremental loading to avoid overwhelming the UI.
Use Efficient Layouts
Layouts directly impact rendering speed. Overusing nested or complex layouts adds unnecessary computation during UI rendering.
Prefer Grid and StackLayout
Use Grid for structured, multi-dimensional layouts and VerticalStackLayout or HorizontalStackLayout for simpler use cases. These are more performant compared to deeply nested combinations.
Avoid Unnecessary Bindings
Data bindings are powerful but can be expensive. Reduce bindings where static values or one-time assignments work just as well.
Optimize Images and Resources
Unoptimized images can bloat your app and slow down both startup and rendering.
Use Scalable Images
Use vector images (like SVGs) wherever possible. In .NET MAUI, consider using FontImageSource for icons to minimize file size and resolution handling.
Leverage ImageSource Smartly
Avoid using images directly from the internet in ImageSource. Cache them locally using libraries like FFImageLoading or MAUI Community Toolkit’s GravatarImage.
Choose the Right Navigation Strategy
Navigation impacts not just UX but also app memory and performance.
Use Shell Navigation
MAUI Shell simplifies navigation with URL-style routing, making transitions faster and less memory-intensive.
await Shell.Current.GoToAsync("//MainPage");
Clear Navigation Stack When Needed
Avoid memory leaks by clearing unused pages or explicitly removing them from the navigation stack after transitions.
Manage Memory and Garbage Collection
Improper memory management can quietly degrade app performance over time.
Unsubscribe from Events
Always unsubscribe from events, especially in custom controls or services. Otherwise, memory leaks will pile up.
myButton.Clicked -= OnClicked;
Use Weak References for Long-Lived Services
For event handlers attached to long-lived services, consider weak references to avoid holding objects in memory unnecessarily.
Monitor Performance with Built-in Tools
Profiling and diagnostics help you identify bottlenecks before your users do.
Use .NET MAUI Performance Diagnostics
Use tools like Visual Studio’s Diagnostic Tools and MAUI’s built-in logging to monitor FPS, memory usage, and CPU cycles.
Integrate App Center or Analytics
For real-world feedback, services like Microsoft App Center, Firebase Performance, or Azure Monitor can track crash logs and performance metrics across platforms.
Handle Platform-Specific Optimizations Gracefully
MAUI lets you write platform-specific code while keeping your UI and logic shared. Use this power wisely.
Use Conditional Compilation
Handle differences in hardware, OS behavior, or performance quirks using compiler directives:
#if ANDROID
// Android-specific optimization
#endif
Extend With Dependency Injection
Use dependency injection to register platform-specific services without cluttering your core logic.
Test on Real Devices — Not Just Emulators
Emulators are convenient, but they rarely mirror real-world performance. For accurate testing:
- Test on low-end Android devices and older iPhones
- Measure startup time, memory usage, and responsiveness under real conditions
- Simulate poor network conditions using tools like Charles Proxy or Android Studio’s Network Profiler
Consider Migration from Xamarin the Right Way
If you’re transitioning from Xamarin.Forms, a clean migration is crucial for performance.
Don’t Just Port — Refactor
Use the opportunity to rethink layout hierarchies, navigation models, and libraries instead of blindly porting everything.
Audit Third-Party Dependencies
Some Xamarin plugins may not be optimized or compatible with MAUI. Replace or refactor them using modern .NET MAUI equivalents or .NET 7+ capabilities.
Final Thoughts: Build Once, Perform Everywhere
By following these best practices, you’re not just building apps — you’re building trust, engagement, and long-term value. Whether you’re launching a new MAUI app or upgrading from Xamarin, prioritizing performance pays off across the board — from user experience to business ROI.
.NET MAUI offers an exciting future for cross-platform development, but it demands smart architecture, conscious coding habits, and a commitment to optimization. Business owners should work with development teams who understand these nuances, while developers must keep performance as a guiding principle from start to finish.
Ready to Build Your Next High-Performance .NET MAUI App?
Partner with experts who’ve mastered .NET MAUI migration, performance tuning, and enterprise-scale app delivery. Let’s turn your vision into a blazing-fast cross-platform reality.
